Java sample code to call the load calculation
web service for container and truck loads
This sample code shows how to pass the shipment
information including the size of the 20FT container and the cargo list from Java
pages to the
remote CubeMaster server and take the calculation back. The web service calculates
the load plan from the shipment information and the load plan is sent to your
computer after the calculation finishes. The client take
the load plan and shows the calculation to
the console of your computer and saves the 3D graphics as GIF picture to a folder at
your computer.
Download full source code of Eclipse project

Download an instruction
for setting the Eclipse for consuming the load calculation web service
 Picture 1>
Result of the Console at your Eclipes
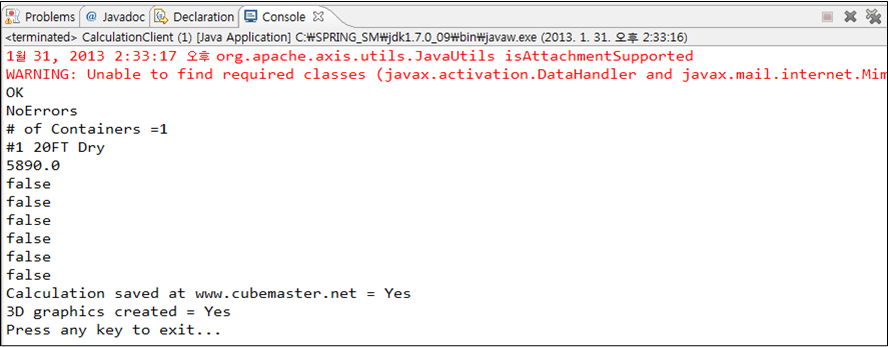
Picture 2> Result of a folder 'C:\Images' at your computer
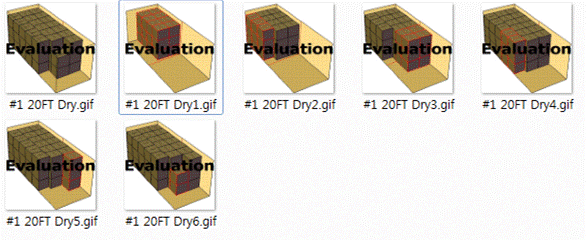
|
|
|
Picture 3> Sample source codes
package org.main;
import java.io.ByteArrayInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.tempuri.*;
import org.apache.axis.encoding.Base64;
import org.datacontract.schemas._2004._07.CubeMasterWebService.*;
public class CalculationClient {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
ICalculationProxy iCalculationProxy = new ICalculationProxy();
//Define account information
//Please replace with the information of your account
//If you are a new user, please create a new free account
//at http://www.cubemaster.net/Subscription/TrialAccount/NewAccount_Form.asp
Account account = new Account();
account.setUserID("TRIAL1");
account.setPassword("0000");
account.setCompany("trials");
//Define options
Options options = new Options();
options.setCalculationSaved(true);
options.setGraphicsCreated(true);
options.setGraphicsImageWidth((short) 300);
options.setGraphicsImageDepth((short) 300);
options.setThumbnailsCreated(true);
options.setThumbnailsImageWidth((short) 300);
options.setThumbnailsImageDepth((short) 300);
//Define shipment
Shipment shipment = iCalculationProxy.getShipment();
shipment.setTitle("New Shipment Sample for Vehicle Load v2");
shipment.setDescription("in C# code");
//Define two cargoes
Cargo NewCargo1[] = new Cargo[1];
NewCargo1[0] = new Cargo();
NewCargo1[0].setName("First Cargo");
NewCargo1[0].setLength(500);
NewCargo1[0].setWidth(500);
NewCargo1[0].setHeight(500);
NewCargo1[0].setQty((long) 40);
NewCargo1[0].setSequence(1);
NewCargo1[0].setGroupName("B");
FilledContainerCollection();
shipment.setCargoes(NewCargo1);
Cargo NewCargo2[] = new Cargo[1];
NewCargo2[0] = new Cargo();
NewCargo2[0].setName("Second Cargo");
NewCargo2[0].setLength(700);
NewCargo2[0].setWidth(800);
NewCargo2[0].setHeight(700);
NewCargo2[0].setQty((long) 56);
NewCargo2[0].setSequence(2);
NewCargo2[0].setGroupName("A");
shipment.setCargoes(NewCargo2);
//Define containers
Container NewContainer[] = new Container[1];
NewContainer[0] = new Container();
NewContainer[0].setContainerType(ContainerContainerTypeEnum.SeaVan);
NewContainer[0].setName("20FT Dry");
NewContainer[0].setLength((double) 5890);
NewContainer[0].setWidth((double) 2330);
NewContainer[0].setHeight((double) 2380);
shipment.setContainers(NewContainer);
//Define Rules
shipment.getRules().setIsWeightLimited(false);
shipment.getRules().setIsSequenceUsed(true);
shipment.getRules().setIsGroupUsed(true);
shipment.getRules().setIsSafeStackingUsed(true);
shipment.getRules().setMinSupportRate((double) 90);
//Run the calculation
LoadPlan loadPlan = iCalculationProxy.run(shipment, account, options);
//Show the return
System.out.println(loadPlan.getStatus());
//Show an error code if any
System.out.println(loadPlan.getCalculationError().toString());
if(loadPlan.getStatus().equals("OK")) {
//Access the results
System.out.println("# of Containers =" + loadPlan.getFilledContainers().length);
InputStream is = null;
OutputStream os = null;
//Show all filled containers
try {
for (FilledContainer container : loadPlan.getFilledContainers()) {
//show the name of the container
System.out.println(container.getName());
System.out.println(container.getLength());
// save the graphics of the container to a folder "c:\\images" at my PC
byte[] bytes = new Base64().decode(container.getBytesImage3D());
byte[] buf = new byte[512];
ByteArrayInputStream as = new ByteArrayInputStream(bytes);
os = new FileOutputStream("c:\\images\\" + container.getName() + ".gif");
while(true){
int cnt = as.read(buf, 0, buf.length);
if(cnt == -1){
break;
}
String str = new String(buf, 0, cnt);
os.write(buf, 0, cnt);
}
//Show all spaces in the container
for (SpaceInContainer Space : container.getSpaces()) {
// if the space if filled, space.IsEmpty is False
System.out.println(Space.getIsEmpty());
if(Space.getIsEmpty() == false) {
//Save the graphics of the load block in the space
bytes = new Base64().decode(Space.getBytesImage3D());
as = new ByteArrayInputStream(bytes);
os = new FileOutputStream("c:\\images\\" + container.getName() +
Space.getSequence() + ".gif");
while(true){
int cnt = as.read(buf, 0, buf.length);
if(cnt == -1){
break;
}
String str = new String(buf, 0, cnt);
os.write(buf, 0, cnt);
}
}
} // End of foreach - SpaceInContainer
} // End of foreach - FilledContainer
} catch (Exception e) {
// TODO: handle exception
} finally { //Resource Cleanup
if(is != null) {
try {
is.close();
os.close();
} catch(IOException e) {
e.printStackTrace();
}
}
} // end of try-catch statement .. for Show all filled containers
} //LoadPlan.Status == "OK"
System.out.println("Calculation saved at www.cubemaster.net = " +
(options.getCalculationSaved() == true? "Yes" : "No"));
System.out.println("3D graphics created = " + (options.getGraphicsCreated() ==
true ? "Yes" : "No"));
System.out.println("Press any key to exit...");
System.exit(0);
} catch (Exception e) {
// TODO: handle exception
}
} // end of main method
private static void FilledContainerCollection() {
// TODO Auto-generated method stub
}
}
|
|
|